The Terminal Problem¶
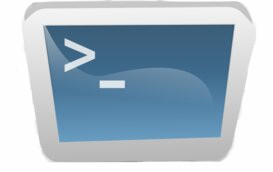
This document discusses serial terminals for use with Mecrisp-Stellaris Forth, their speed, different options and compromises.
Background¶
Compared to fast modern embedded development systems such as C, traditional Forth has a common problem, in the simplest case it is painfully slow serial terminals resulting in slow, painful development.
Never fear tho, this can be fixed so uploading is so fast you can’t read the text on the terminal screen, resulting in a development system that’s much faster than the latest C IDE flavor of the day. There are many choices of solution depending on your level of programmer and electronics skill, choice of MCU etc.
Terminal¶
Forth has to have a way to accept user commands for its much valued user interactivity and to allow uploading source code files during embedded development. As the source is compiled on the MCU as it’s uploaded, it makes sense to have the fastest upload method possible.
It normally does this via a serial terminal hosted on the users PC which provides a Command Line Interface for the user as illustrated below.
Mecrisp-Stellaris RA 2.5.4 with M0 core for STM32F051 by Matthias Koch
ok.
Mecrisp-Stellaris Terminal Driver¶
The default Mecrisp-Stellaris USART driver does not include any data handshaking so that it will always work, tho various models may feature it as a compile time option, (usually user supplied). Matthias Koch will generally accept a tested and working user submission providing it has built in compile time switch, disabled by default. Your name will be added to the contributor list if you do so.
Hardware Flow Control¶
This is the traditional data comms method for maximum speed. The Forth MCU signals the PC terminal to stop or start sending data via control of the RTS (Request To Send) signal. This requires a extra wire between MCU and serial dongle.
RTS handshaking is the most robust but not the easiest choice of solution to use, but then it’s not very difficult as it only involves a extra signal, wire and possibly a Kernel option recompilation or even the addition of some new Kernel supporting code. If your hardware isn’t already set up for this and you don’t do electronics and can’t solder etc, this may be a bridge too far, so software only solutions may have greater appeal.
Enabling Hardware Flow Control¶
Where supported.
“.equ rtscts, 1” is added to mecrisp-stellaris-2.6.1/mecrisp-stellaris-source/stm32fxxxxx/mecrisp-stellaris-stm32fxxxxx.s and the kernel is then recompiled.
@ -----------------------------------------------------------------------------
@ Swiches for capabilities of this chip
@ -----------------------------------------------------------------------------
.equ rtscts, 1
... other kernel options
Example Hardware Flow Control Code¶
This is my early contribution for the STM32F051. Basically it configures some GPIO pins, sets the Alternate Function for them and enables RTS and CTS (Clear To Send isn’t used as the PC is always fast enough, but the CTS pin on the MCU will need to be taken to logic level LOW with a wire as its so sensitive and may seem to cause lockups otherwise).
It’s very simple and works every time, however it also means that the serial device must be able to talk RTS/CTS along with the terminal client on the PC.
Partial code snippet taken from mecrisp-stellaris-2.6.1/mecrisp-stellaris-source/stm32f051-ra/terminal.s This will vary for a different chip, different pinouts, different USART etc. This code is written in ARM Thumb1 Assembly as is Mecrisp-Stellaris.
.equ GPIOA_BASE , 0x48000000
.equ GPIOA_MODER , GPIOA_BASE + 0x00
.equ GPIOA_OTYPER , GPIOA_BASE + 0x04
.equ GPIOA_OSPEEDR , GPIOA_BASE + 0x08
.equ GPIOA_PUPDR , GPIOA_BASE + 0x0C
.equ GPIOA_IDR , GPIOA_BASE + 0x10
.equ GPIOA_ODR , GPIOA_BASE + 0x14
.equ GPIOA_BSRR , GPIOA_BASE + 0x18
.equ GPIOA_LCKR , GPIOA_BASE + 0x1C
.equ GPIOA_AFRL , GPIOA_BASE + 0x20
.equ GPIOA_AFRH , GPIOA_BASE + 0x24
.equ GPIOA_BRR , GPIOA_BASE + 0x28
.equ USART1_BASE , 0x40013800
.equ USART1_CR1 , USART1_BASE + 0x00
.equ USART1_CR2 , USART1_BASE + 0x04
.equ USART1_CR3 , USART1_BASE + 0x08
.equ USART1_BRR , USART1_BASE + 0x0C
.equ USART1_GTPR , USART1_BASE + 0x10
.equ USART1_RTOR , USART1_BASE + 0x14
.equ USART1_RQR , USART1_BASE + 0x18
.equ USART1_ISR , USART1_BASE + 0x1C
.equ USART1_ICR , USART1_BASE + 0x20
.equ USART1_RDR , USART1_BASE + 0x24
.equ USART1_TDR , USART1_BASE + 0x28
.ifdef rtscts
@ Set PORTA pin modes: PA9 (TX-OUTPUT)-SF, PA10 (RX-INPUT)-SF, PA11 (CTS-INPUT)-SF, PA12 (RTS-OUTPUT)-SF, PA13 (SWDIO)-AF, PA14 (SWCLK)-AF
@ gpioa_moder. GPIOA_MODER (read-write) $2AA80000
@ 15|14|13|12|11|10|09|08|07|06|05|04|03|02|01|00
@ 00 10 10 10 10 10 10 00 00 00 00 00 00 00 00 00
ldr r1, = GPIOA_MODER
ldr r0, = 0x2AA80000
str r0, [r1]
.else
@ Set PORTA pins 9 and 10 in alternate function mode
ldr r1, = GPIOA_MODER
ldr r0, = 0x28280000 @ 2800 0000 is Reset value for Port A, and switch PA9 and PA10 to alternate function
str r0, [r1]
.endif
.ifdef rtscts
@ set PORTA Alternate Functions: PA9 (TX), PA10 (RX), PA11 (CTS), PA12 (RTS) all to AF1
ldr r1, = GPIOA_AFRH
ldr r0, = 0x011110 @ Alternate function 1 for TX, RX, CTS and RTS pins of USART1 on PORTA (tp)
str r0, [r1]
.else
@ Set alternate function 1 to enable USART1 pins on Port A
ldr r1, = GPIOA_AFRH
ldr r0, = 0x110 @ Alternate function 1 for TX and RX pins of USART1 on PORTA
str r0, [r1]
.endif
.ifdef rtscts
@ Enable RTS/CTS flow control (tp)
ldr r1, = USART1_CR3
ldr r0, = 0x300 @ Enable RTS flow control, RTSE (bit 8) = 1, and CTSE (bit 9) = 1
str r0, [r1]
.endif
Terminals: Slow to Fast¶
Slow
Serial at 115200 Baud with a end of line delay, 20 to 200 ms
Serial at 115200 Baud with flow control, either hardware of software.
Serial at 46800 Baud and over, with flow control.
USB which provides a Virtual Comms Device. This will include flow control and operate at the fastest stable serial rate
Ethernet (not discussed here) other than mentioning that at least one Mecrisp-Stellaris port features this. Example: tm4c1294-ra which has a beautiful Ethernet driver featuring ca DHCP client.
Fast
Terminals: PC Hosted¶
There are many types depending on OS, FLOSS/nonfree, and so on. Picom is a simple and reliable Unix favorite which is easy to use and slow or fast depending on its connection strategy.
Uploaders¶
Terminals like Picocom use a uploader application which feeds Forth source file contents into the terminal and hence to the Forth Target. The standard uploader, included in the Picom releases is named ascii-xfr and via Picom allow none, xon/xoff, or RTS data handshaking via user configuration.